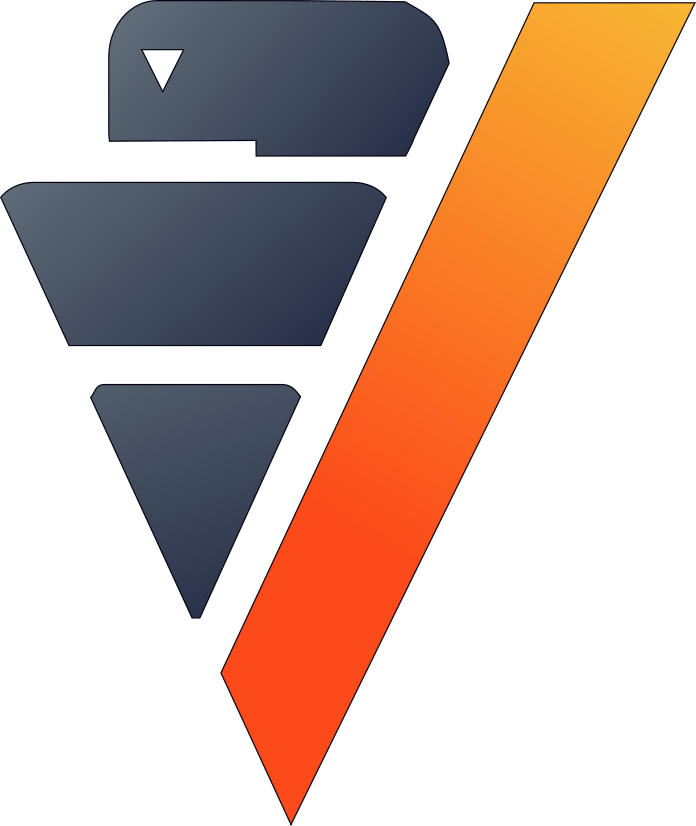
VerticaPy
Example: Methods in an Unsupervised Model¶
In this example, we use the 'Iris' dataset to demonstrate the methods available to unsupervised models.
from verticapy.datasets import load_iris
iris = load_iris()
display(iris)
Let's create a k-means model to segment the data into clusters.
from verticapy.learn.cluster import KMeans
model = KMeans("public.KMeans_iris", n_cluster = 3)
model.fit("public.iris", ["PetalWidthCm", "PetalLengthCm"])
Fitting the model creates new model attributes.
model.X
model.input_relation
These attributes will be used when invoking the different model abstractions.
Models have many useful attributes. For a k-means model, the 'clustercenters' and 'metrics_' attributes can give you an idea of the quality of the model.
model.cluster_centers_
model.metrics_
You can use the 'get_attr' method to view other attributes.
model.get_attr()
Let's look at the SQL query for our model.
display(model.deploySQL())
You can add the prediction to your vDataFrame.
model.predict(iris, name = "pred_Species")
Let's examine the prediction with a histogram and graph.
iris.hist(["Species", "pred_Species"])
model.plot()
Some algorithms, like DBSCAN and LOF are constructed with SQL queries.
from verticapy.learn.cluster import DBSCAN
model = DBSCAN("public.DBSCAN_iris")
model.fit("public.iris", ["PetalWidthCm", "PetalLengthCm"])
from verticapy.learn.neighbors import LocalOutlierFactor
model2 = LocalOutlierFactor("public.LocalOutlierFactor_iris")
model2.fit("public.iris", ["PetalWidthCm", "PetalLengthCm"])
These models store the results in a table. We can make predictions with this model using the 'predict' method.
model.predict()
model2.predict()
Let's plot our model.
model.plot()
model2.plot()